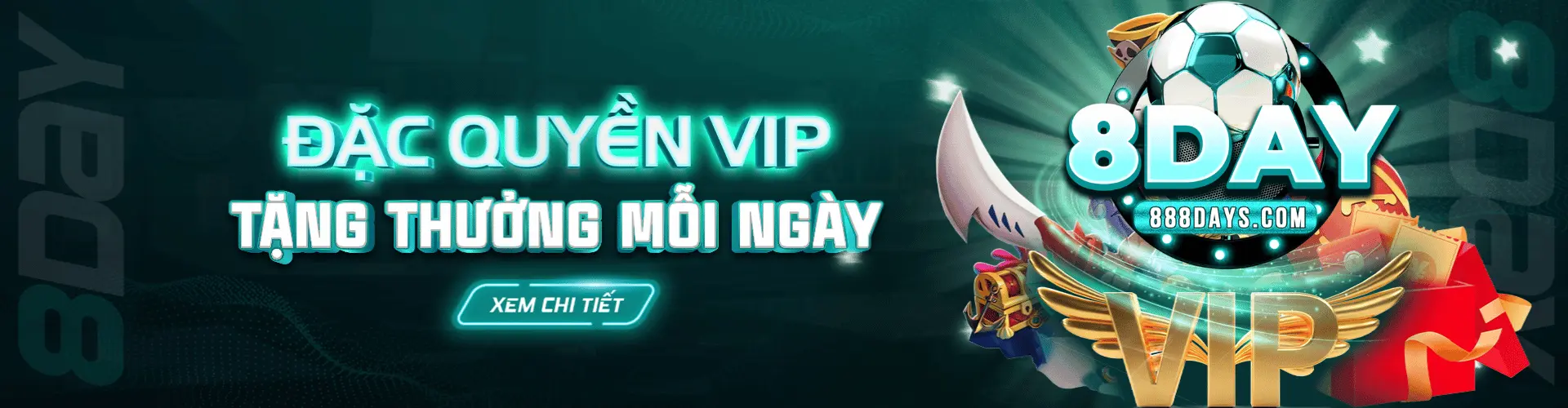
8DAY – Trang Chủ Đăng Nhập Chính Thức Không Bị Chặn
8DAY – 8DAY COM Nhà cái đẳng cấp số 1 châu Á mang đến trải nghiệm cá cược đỉnh cao với giao diện hiện đại, tỉ lệ cược hấp dẫn và dịch vụ khách hàng chuyên nghiệp, đảm bảo sự an tâm và thú vị cho người chơi mọi lúc, mọi nơi. Tham gia ngay 8DAY nhận ưu đãi lên đến 888k!